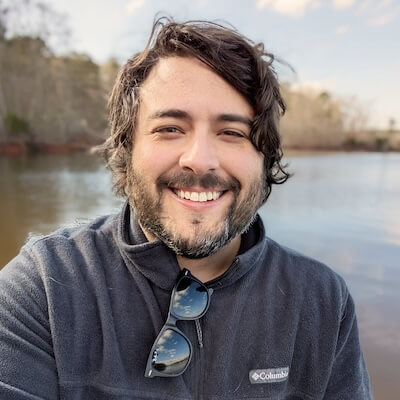
Chris Arter
Senior Software Engineer
Hey there! 👋 I'm a senior software engineer with over a decade of experience based in North Carolina, USA. I'm a Laravel core contributor, and I'm passionate about problem solving, community building, and learning new things. Above all else, I'm a Dad to two amazing kids, and husband to an amazing wife.